What Is JavaScript?
What is JavaScript programming language? JavaScript is a high-level, interpreted programming language. It is a language that is also characterized as dynamic, weakly typed, prototype-based, and multi-paradigm. Alongside HTML and CSS, JavaScript is one of the three core technologies of the World Wide Web. JavaScript enables interactive web pages and thus is an essential part of web applications. The vast majority of websites use it for client-side page processing.
JavaScript: Explained
JavaScript is a programming language that was created in 1995. It was designed to make web pages more interactive and allow for more complex applications. JavaScript is a versatile language that can be used for many different purposes. Today, JavaScript is one of the most popular programming languages in the world.
What Can You Do With JavaScript?
JavaScript is a versatile language that allows you to build web applications, games, and more. Here are some things you can do with JavaScript:
- Build web applications: You can use JavaScript to add interactivity to your website or application. For example, you can create forms, validate user input, and display dynamic content.
- Build games: Games are a popular use for JavaScript. You can use JavaScript to create 2D and 3D games, add sound and graphics, and create levels and characters.
- Create animations: You can use JavaScript to animate elements on your webpage or app. This could include things like scrolling text or images, fading elements in and out, or moving elements around the screen. You can even create animation effects for mobile apps.
- Create regular expressions Regular expressions are a powerful way to search and manipulate text. You can use JavaScript to create regular expressions that find patterns in text, and parse text into different parts.
How To Use JavaScript: Where To Put JS Code And How To Write It
JavaScript is a programming language that can be used to add interactivity to websites.
Where to put JS code: You can put JavaScript code in the <head> or <body> section of your HTML document.
How to write it: When writing JavaScript code, you will use the <script> tag. This tag tells the browser that you are using a scripting language.
JavaScript Syntax: Basic Rules For Writing JS Code
JavaScript syntax is the basic rule for writing JS code. JS code must be written in a certain way in order for the browser to understand it. The main rule is that all JS code must be placed inside of <script> tags. Other than that, there are no strict rules about how JS code must be written. However, there are some best practices that should be followed in order to make your code more readable and maintainable.
Some Of The Basic Rules For JavaScript Syntax Include:
• All JS code must be placed inside of <script> tags.
• JS code can be written either in an external .js file or directly inline within the HTML document.
• Variable names can contain letters, numbers, underscores, and dollar signs – but they cannot start with a number.
Variables: What Variables Are And How To Use Them In JS
JavaScript variables are like containers that store data values. In other programming languages, variables are often called “objects.” A variable can hold any data type, including numbers, strings, and even other objects.
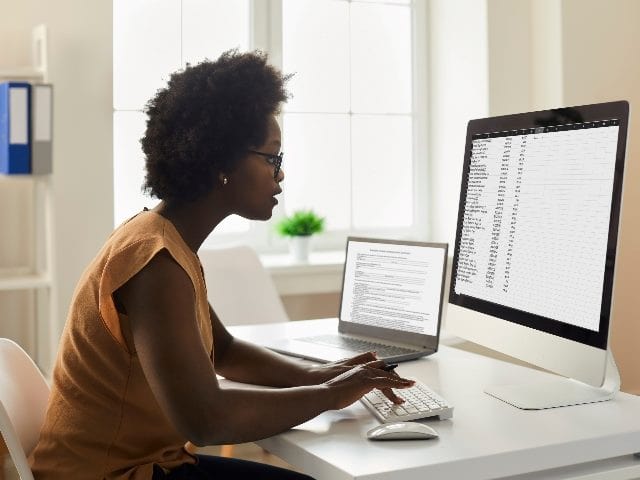
You can create a variable by using the var keyword, followed by the variable name and an equal sign (=). The value of the variable can be assigned on the same line after the equal sign, or on a separate line:
var myName = “John”;
myName = “Jane”;
You can also declare multiple variables in one statement by separating each variable name with a comma:
var firstName = “John”, lastName = “Doe”;
If you try to use a variable that has not been declared, JavaScript[1] will throw an error. Therefore, it’s important to always declare your variables before using them.
Operators: Different Types Of Operators Available In JS
An operator is a symbol that tells the JavaScript interpreter to perform a certain operation. The different types of operators available in JS are:
Arithmetic Operators: These include the basic math operators like +, -, *, /, and %.
Assignment Operators: These include the = operator, which assigns a value to a variable. There are also compound assignment operators like +=, -=, *=, and /=, which perform the arithmetic operation and assignment in one step.
Comparison Operators: These compare two values and return either true or false. The most common comparison operator is == (equal to), but there are also != (not equal to), === (strictly equal to), !
Conclusion
JavaScript is a versatile scripting language that enables you to create interactive web pages. This article has discussed the basics of JavaScript, including its syntax, variables, operators, and functions. With a little practice, you should be able to use JavaScript to add interactivity to your web pages.